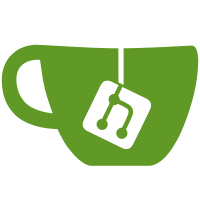
On a recent build I ran in to the following error messages: Wed May 3 14:31:43 2017 /sbin/ip -6 addr add 2001:db8:0:4::1/64 dev tun0 Wed May 3 14:31:43 2017 Linux ip -6 addr add failed: external program exited with error status: 2 This appears to be do to the fact that somewhere something defaulted the kernel in the container to disable IPv6. Not sure if this is my host or the docker daemon. Re-enable it explicitly for now until Docker gets it's IPv6 act together.
100 lines
2.8 KiB
Bash
Executable File
100 lines
2.8 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
#
|
|
# Run the OpenVPN server normally
|
|
#
|
|
|
|
if [ "$DEBUG" == "1" ]; then
|
|
set -x
|
|
fi
|
|
|
|
set -e
|
|
|
|
cd $OPENVPN
|
|
|
|
# Build runtime arguments array based on environment
|
|
USER_ARGS=("${@}")
|
|
ARGS=()
|
|
|
|
# Checks if ARGS already contains the given value
|
|
function hasArg {
|
|
local element
|
|
for element in "${@:2}"; do
|
|
[ "${element}" == "${1}" ] && return 0
|
|
done
|
|
return 1
|
|
}
|
|
|
|
# Adds the given argument if it's not already specified.
|
|
function addArg {
|
|
local arg="${1}"
|
|
[ $# -ge 1 ] && local val="${2}"
|
|
if ! hasArg "${arg}" "${USER_ARGS[@]}"; then
|
|
ARGS+=("${arg}")
|
|
[ $# -ge 1 ] && ARGS+=("${val}")
|
|
fi
|
|
}
|
|
|
|
# set up iptables rules and routing
|
|
# this allows rules/routing to be altered by supplying this function
|
|
# in an included file, such as ovpn_env.sh
|
|
function setupIptablesAndRouting {
|
|
iptables -t nat -C POSTROUTING -s $OVPN_SERVER -o $OVPN_NATDEVICE -j MASQUERADE || {
|
|
iptables -t nat -A POSTROUTING -s $OVPN_SERVER -o $OVPN_NATDEVICE -j MASQUERADE
|
|
}
|
|
for i in "${OVPN_ROUTES[@]}"; do
|
|
iptables -t nat -C POSTROUTING -s "$i" -o $OVPN_NATDEVICE -j MASQUERADE || {
|
|
iptables -t nat -A POSTROUTING -s "$i" -o $OVPN_NATDEVICE -j MASQUERADE
|
|
}
|
|
done
|
|
}
|
|
|
|
|
|
addArg "--config" "$OPENVPN/openvpn.conf"
|
|
|
|
source "$OPENVPN/ovpn_env.sh"
|
|
|
|
mkdir -p /dev/net
|
|
if [ ! -c /dev/net/tun ]; then
|
|
mknod /dev/net/tun c 10 200
|
|
fi
|
|
|
|
if [ -d "$OPENVPN/ccd" ]; then
|
|
addArg "--client-config-dir" "$OPENVPN/ccd"
|
|
fi
|
|
|
|
# When using --net=host, use this to specify nat device.
|
|
[ -z "$OVPN_NATDEVICE" ] && OVPN_NATDEVICE=eth0
|
|
|
|
# Setup NAT forwarding if requested
|
|
if [ "$OVPN_DEFROUTE" != "0" ] || [ "$OVPN_NAT" == "1" ] ; then
|
|
# call function to setup iptables rules and routing
|
|
# this allows rules to be customized by supplying
|
|
# a replacement function in, for example, ovpn_env.sh
|
|
setupIptablesAndRouting
|
|
fi
|
|
|
|
# Use a hacky hardlink as the CRL Needs to be readable by the user/group
|
|
# OpenVPN is running as. Only pass arguments to OpenVPN if it's found.
|
|
if [ -r "$EASYRSA_PKI/crl.pem" ]; then
|
|
if [ ! -r "$OPENVPN/crl.pem" ]; then
|
|
ln "$EASYRSA_PKI/crl.pem" "$OPENVPN/crl.pem"
|
|
chmod 644 "$OPENVPN/crl.pem"
|
|
fi
|
|
addArg "--crl-verify" "$OPENVPN/crl.pem"
|
|
fi
|
|
|
|
ip -6 route show default 2>/dev/null
|
|
if [ $? = 0 ]; then
|
|
echo "Enabling IPv6 Forwarding"
|
|
# If this fails, ensure the docker container is run with --privileged
|
|
# Could be side stepped with `ip netns` madness to drop privileged flag
|
|
|
|
sysctl -w net.ipv6.conf.all.disable_ipv6=0 || echo "Failed to enable IPv6 support"
|
|
sysctl -w net.ipv6.conf.default.forwarding=1 || echo "Failed to enable IPv6 Forwarding default"
|
|
sysctl -w net.ipv6.conf.all.forwarding=1 || echo "Failed to enable IPv6 Forwarding"
|
|
fi
|
|
|
|
echo "Running 'openvpn ${ARGS[@]} ${USER_ARGS[@]}'"
|
|
exec openvpn ${ARGS[@]} ${USER_ARGS[@]}
|