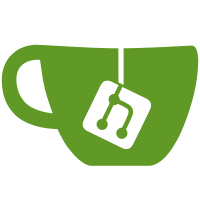
subrepo: subdir: "deps/clap-juce-extensions/clap-libs/clap" merged: "3189bdfaf" upstream: origin: "https://github.com/free-audio/clap" branch: "main" commit: "3189bdfaf" git-subrepo: version: "0.4.3" origin: "https://github.com/ingydotnet/git-subrepo.git" commit: "2f68596"
56 lines
1.3 KiB
C
56 lines
1.3 KiB
C
#pragma once
|
|
|
|
#include "../../plugin.h"
|
|
|
|
// This extension can be used to specify the channel mapping used by the plugin.
|
|
|
|
static CLAP_CONSTEXPR const char CLAP_EXT_AMBISONIC[] = "clap.ambisonic.draft/0";
|
|
|
|
static CLAP_CONSTEXPR const char CLAP_PORT_AMBISONIC[] = "ambisonic";
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
enum {
|
|
// FuMa channel ordering
|
|
CLAP_AMBISONIC_FUMA = 0,
|
|
|
|
// ACN channel ordering
|
|
CLAP_AMBISONIC_ACN = 1,
|
|
};
|
|
|
|
enum {
|
|
CLAP_AMBISONIC_NORMALIZATION_MAXN = 0,
|
|
CLAP_AMBISONIC_NORMALIZATION_SN3D = 1,
|
|
CLAP_AMBISONIC_NORMALIZATION_N3D = 2,
|
|
CLAP_AMBISONIC_NORMALIZATION_SN2D = 3,
|
|
CLAP_AMBISONIC_NORMALIZATION_N2D = 4,
|
|
};
|
|
|
|
typedef struct clap_ambisonic_info {
|
|
uint32_t ordering;
|
|
uint32_t normalization;
|
|
} clap_ambisonic_info_t;
|
|
|
|
typedef struct clap_plugin_ambisonic {
|
|
// Returns true on success
|
|
// [main-thread]
|
|
bool (*get_info)(const clap_plugin_t *plugin,
|
|
bool is_input,
|
|
uint32_t port_index,
|
|
clap_ambisonic_info_t *info);
|
|
|
|
} clap_plugin_ambisonic_t;
|
|
|
|
typedef struct clap_host_ambisonic {
|
|
// Informs the host that the info have changed.
|
|
// The info can only change when the plugin is de-activated.
|
|
// [main-thread]
|
|
void (*changed)(const clap_host_t *host);
|
|
} clap_host_ambisonic_t;
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|